Proper singleton implementation in C#
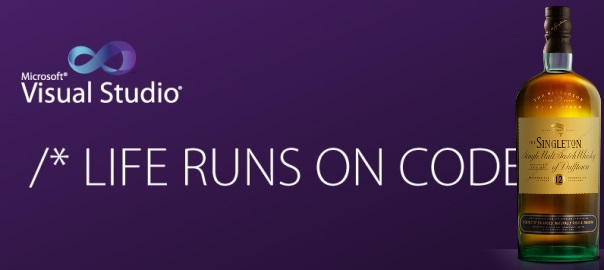
Taken from: http://msdn.microsoft.com/en-us/library/ff650316.aspx
public class Singleton { private static Singleton instance; private Singleton() {} public static Singleton Instance { get { if (instance == null) { instance = new Singleton(); } return instance; } } }
Static initialization
public sealed class Singleton { private static readonly Singleton instance = new Singleton(); private Singleton(){} public static Singleton Instance { get { return instance; } } }
Multithreaded singleton
public sealed class Singleton { private static volatile Singleton instance; private static object syncRoot = new Object(); private Singleton() {} public static Singleton Instance { get { if (instance == null) { lock (syncRoot) { if (instance == null) instance = new Singleton(); } } return instance; } } }
Test program; on first form you have two buttons, one which sets the amount of singleton, and second button which opens up another form which then sets the amount again. Once returned to the first form, the value updates to the one set on second form.
//Form1.cs public partial class Form1 : Form { myClass mc; public Form1() { InitializeComponent(); mc = myClass.Instance; } private void button1_Click(object sender, EventArgs e) { mc.iznos = 10; updateUI(); } public void updateUI() { label1.Text = mc.iznos.ToString(); } private void button2_Click(object sender, EventArgs e) { Form2 f2 = new Form2(); f2.ShowDialog(); } private void Form1_Activated(object sender, EventArgs e) { updateUI(); } }
//Form2.cs public partial class Form2 : Form { myClass mc; public Form2() { InitializeComponent(); mc = myClass.Instance; } private void Form2_Load(object sender, EventArgs e) { mc.iznos = 5; updateUI(); } public void updateUI() { label1.Text = mc.iznos.ToString(); } }
public class myClass{ private static readonly myClass _instance = new myClass(); private myClass(){} public static myClass Instance { get { return _instance; } } public int iznos { get; set; } }
I found your blog to be very informative and interesting. On similar lines you can also check out http://crbtech.in/Dot-Net-Training/singleton-c-pattern-anti-pattern/ which is also a very good blog on this very topic. Request you to continue writing on varied topics as we would like to read.
Thank you, Kailas.