Wait for click before starting the game in Phaser
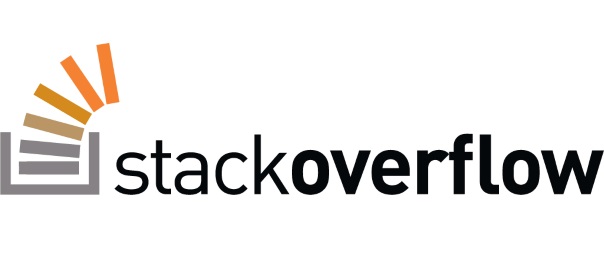
I’m a big fan of Stack Overflow and I tend to contribute regularly (am currently in the top 0.X%). In this category (stackoverflow) of posts I will will be posting my top rated questions and answers. This, btw, is allowed as explained in the meta thread here.
My question was:
The JSFiddle demo is here, but am pasting the code below also. My question is simple (though I can’t seem to find any example in how to realize this): right now if you start the game in JSFiddle you will see that the rectangle immediately “falls” down. I would somehow like the game to start when I click with the mouse (basically that nothing happens before the first click of a mouse) – any points on how to accomplish this?
JS:
// Initialize Phaser, and creates a 400x490px game var game = new Phaser.Game(400, 490, Phaser.AUTO, 'game_div'); var game_state = {}; // Creates a new 'main' state that wil contain the game game_state.main = function () {}; game_state.main.prototype = { preload: function () { // Change the background color of the game this.game.stage.backgroundColor = '#71c5cf'; // Load the bird sprite this.game.load.image('bird', 'https://lh6.googleusercontent.com/Xrz0PnR6Ezg5_k5zyFKxGv0LzehAP9SMj_ga3qQzIF4JAfv8xHm7TxfliwtBD8ihfw=s190'); this.game.load.image('pipe', 'https://lh4.googleusercontent.com/RSMNhJ3KY4Xl0PQpUf6I9EayOdLhvOKKV9QV7_BXXYVedPy0oMNRFKANW14xV76NDA=s190'); }, create: function () { // Display the bird on the screen this.bird = this.game.add.sprite(100, 245, 'bird'); // Add gravity to the bird to make it fall this.bird.body.gravity.y = 1000; // Call the 'jump' function when the spacekey is hit var space_key = this.game.input.keyboard.addKey(Phaser.Keyboard.SPACEBAR); space_key.onDown.add(this.jump, this); this.pipes = game.add.group(); this.pipes.createMultiple(20, 'pipe'); this.timer = this.game.time.events.loop(1500, this.add_row_of_pipes, this); this.score = 0; var style = { font: "30px Arial", fill: "#ffffff" }; this.label_score = this.game.add.text(20, 20, "0", style); }, update: function () { // If the bird is out of the world (too high or too low), call the 'restart_game' function this.game.physics.overlap(this.bird, this.pipes, this.restart_game, null, this); if (this.bird.inWorld == false) this.restart_game(); }, // Make the bird jump jump: function () { // Add a vertical velocity to the bird this.bird.body.velocity.y = -350; }, // Restart the game restart_game: function () { // Start the 'main' state, which restarts the game this.game.time.events.remove(this.timer); this.game.state.start('main'); }, add_one_pipe: function (x, y) { // Get the first dead pipe of our group var pipe = this.pipes.getFirstDead(); // Set the new position of the pipe pipe.reset(x, y); // Add velocity to the pipe to make it move left pipe.body.velocity.x = -200; // Kill the pipe when it's no longer visible pipe.outOfBoundsKill = true; }, add_row_of_pipes: function () { this.score += 1; this.label_score.content = this.score; var hole = Math.floor(Math.random() * 5) + 1; for (var i = 0; i < 8; i++) if (i != hole && i != hole + 1) this.add_one_pipe(400, i * 60 + 10); }, }; // Add and start the 'main' state to start the game game.state.add('main', game_state.main); game.state.start('main');
CSS:
#game_div { width: 400px; margin: auto; margin-top: 50px; }
HTML:
<div id="game_div"></div>
I found the answer to this one myself:
So, to not leave this question unanswered and to help further readers, here is the link to the updated jsFiddle where I solved my initial problem, and below is the code from jsFiddle.
However, if one will be interested I suggest taking a look at the freely available code on GitHub which uses game states and has a better structured code.
jsFiddle
CSS:
#game_div { width: 400px; margin: auto; margin-top: 50px; }HTML:
<div id="game_div"></div>JS:
// Initialize Phaser, and creates a 400x490px game var game = new Phaser.Game(400, 490, Phaser.AUTO, 'game_div'); var game_state = {}; var gameStarted = false; // Creates a new 'main' state that wil contain the game game_state.main = function () {}; game_state.main.prototype = { game_start: function(){ if (!gameStarted){ this.bird.body.gravity.y = 1000; this.timer = this.game.time.events.loop(1500, this.add_row_of_pipes, this); this.label_start.content = ""; } gameStarted = true; }, preload: function () { // Change the background color of the game this.game.stage.backgroundColor = '#71c5cf'; // Load the bird sprite this.game.load.image('bird', 'https://lh6.googleusercontent.com/Xrz0PnR6Ezg5_k5zyFKxGv0LzehAP9SMj_ga3qQzIF4JAfv8xHm7TxfliwtBD8ihfw=s190'); this.game.load.image('pipe', 'https://lh4.googleusercontent.com/RSMNhJ3KY4Xl0PQpUf6I9EayOdLhvOKKV9QV7_BXXYVedPy0oMNRFKANW14xV76NDA=s190'); }, create: function () { // Display the bird on the screen this.bird = this.game.add.sprite(100, 245, 'bird'); // Add gravity to the bird to make it fall // Call the 'jump' function when the spacekey is hit this.game.input.onDown.add(this.game_start, this); this.pipes = game.add.group(); this.pipes.createMultiple(20, 'pipe'); this.score = 0; var style = { font: "30px Arial", fill: "#ffffff" }; this.label_score = this.game.add.text(20, 20, "0", style); this.label_start = this.game.add.text(35, 180, "Click to start the show", style); game.input.onDown.add(this.jump, this); }, update: function () { // If the bird is out of the world (too high or too low), call the 'restart_game' function this.game.physics.overlap(this.bird, this.pipes, this.restart_game, null, this); if (this.bird.inWorld == false) this.restart_game(); }, // Make the bird jump jump: function () { // Add a vertical velocity to the bird this.bird.body.velocity.y = -350; }, // Restart the game restart_game: function () { // Start the 'main' state, which restarts the game this.game.time.events.remove(this.timer); this.game.state.start('main'); gameStarted=false; }, add_one_pipe: function (x, y) { // Get the first dead pipe of our group var pipe = this.pipes.getFirstDead(); // Set the new position of the pipe pipe.reset(x, y); // Add velocity to the pipe to make it move left pipe.body.velocity.x = -200; // Kill the pipe when it's no longer visible pipe.outOfBoundsKill = true; }, add_row_of_pipes: function () { this.score += 1; this.label_score.content = this.score; var hole = Math.floor(Math.random() * 5) + 1; for (var i = 0; i < 8; i++) if (i != hole && i != hole + 1) this.add_one_pipe(400, i * 60 + 10); }, }; // Add and start the 'main' state to start the game game.state.add('main', game_state.main); game.state.start('main');
Leave a Comment