This is How Your Parents Used CSS and JavaScript
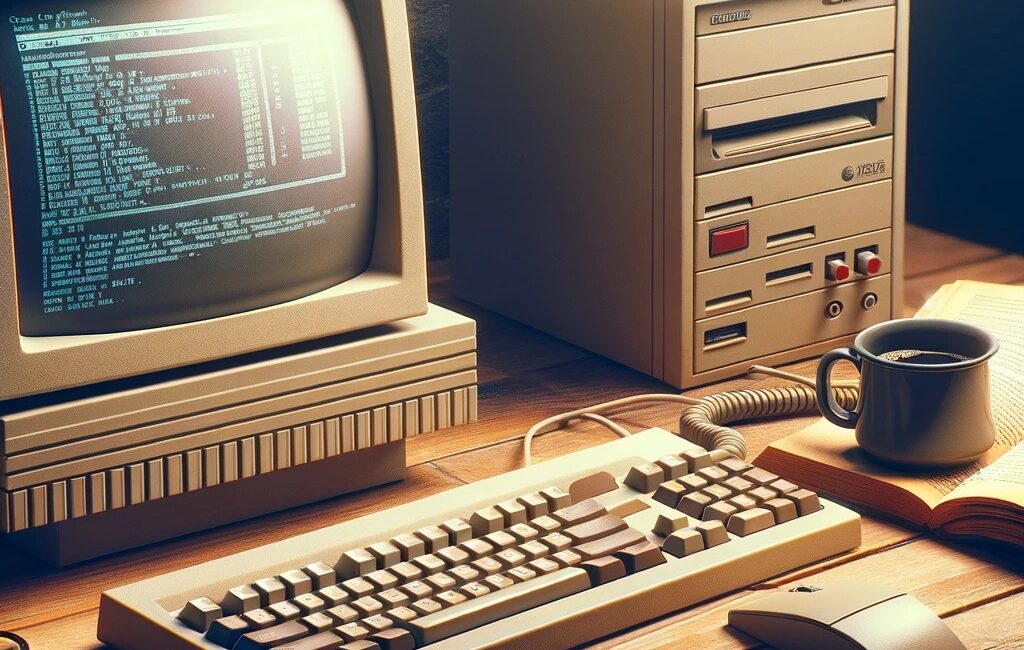
TL;DR
This is the second part of the "This is How Your Parents Used to Build Websites". The first blog post is here.
Now, if you thought our HTML journey was exciting, buckle up! We’re about to dive into the world of CSS and JavaScript. These are the tools that will transform your static HTML pages into vibrant, interactive experiences.
Ready? Let’s go!
Part 1: CSS – Making Your Website Stylish
Basic Concepts of CSS
This is how a simple CSS statement would look like: p { color: blue};
.
There are three basic concepts of CSS:
- Selectors:
- Think of them as the ‘who’ in styling.
- For example,
p { }
targets all<p>
elements.
- Properties and Values:
- The ‘what’ and ‘how’. They go withing the
{}
brackets. - Like
color: blue;
– this tells the paragraph text to be blue.
- The ‘what’ and ‘how’. They go withing the
Incorporating CSS into HTML
There are three ways you can add CSS into HTML:
- Inline Styles: Directly in an HTML tag, like
<p style="color: blue;">
. - Internal Styles: Inside the
<style>
tag in the HTML head. - External Stylesheets: A separate
.css
file, linked in the HTML head with<link rel="stylesheet" href="style.css">
.
Exploring Common CSS Properties
Color and Fonts
body { color: #333; font-family: 'Arial', sans-serif; }
Font Size, Weight, and Style
p {
font-size: 16px;
font-weight: bold;
font-style: italic;
}
Text Alignment and Decoration
h1 {
text-align: center;
text-decoration: underline;
}
Margin and Padding
.content {
margin: 20px;
padding: 15px;
}
Borders
.box {
border: 2px solid #000000; /* Solid black border */
border-radius: 10px; /* Rounded corners */
}
Styling Links
-
Hover Effect
a:hover { color: #ff0000; /* Red color on hover */ text-decoration: none; }
-
Link Visited State
a:visited { color: #800080; /* Purple for visited links */ }
Layout with Flexbox
display: flex;
enables a flexible box layout.- There are games that explain flexbox, so if you’re curious go check them out.
Responsive Design Basics
In order to adapt the site to various screen sizes and make sure it looks good on an iPhone as well as on your laptop, we can use the so-called media queries:
@media (max-width: 600px) { body { background-color: lightblue; }}
This changes the background color on screens smaller than 600px.
Advanced Selectors
-
Pseudo-classes
a:hover { color: red; }
changes link color on hover -
CSS Transitions
transition: background-color 0.5s ease;
-
CSS Animations
@keyframes example { from {background-color: red;} to {background-color: yellow;} } div { animation-name: example; animation-duration: 4s; }
Advanced CSS
If you’re curious enough, please explore SASS (CSS with superpowers) on your own.
Part 2: JavaScript – Bringing Interactivity to Your Website
JavaScript is the scripting language that lets you create dynamic content.
Basic Syntax and Concepts
Variables
let message = 'Hello, world!';
– with this we defined the variable called ‘message’ and saved in it the string ‘Hello, world!’.
You may come across the keyword var
, but that was an old keyword that is no longer suggested to be used because of the unintended consequences.
Functions
function greet(name) {
alert('Hello, ' + name);
}
With this we created a function called greet
that takes a parameter called name
and outputs Hello name
via an alert (OFC, name is replaced with whatever you pass into the function as a parameter).
Events
<button onclick="greet('Alice')">Greet</button>
With onclick
we define what function is going to be run when the button is clicked.
DOM Manipulation
-
Change text
document.getElementById('myText').innerHTML = 'New Text';
-
A button that changes a theme color
<button onclick="changeColor()">Change Theme</button> <script> function changeColor() { document.body.style.backgroundColor = 'lightblue'; } </script>
-
Changing styles on hover using JavaScript
document.getElementById('myElement').onmouseover = function() { this.style.color = 'red'; };
-
Interactive portfolio enhancements
document.getElementById('project1').onmouseover = function() { this.style.transform = 'scale(1.1)'; }; document.getElementById('project1').onmouseout = function() { this.style.transform = 'scale(1)'; };
-
Handling a click event
document.getElementById('myButton').addEventListener('click', function() { alert('Button clicked!'); });
Advanced topics
Using fetch
to load data
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
I wrote a three series blog post on doing so-called AJAX calls (fetching data from some API), check them out here:
- Making AJAX calls in pure JavaScript
- Making AJAX calls using jQuery
- Making AJAX calls using the Fetch API
JavaScript ES6
There are some awesome things that have been added to the ‘new’ flavor of JavaScript, called ES6. I won’t go into the details here, but use Google (or ChatGPT, or Bard, or …) and look into these topics:
- Arrow Functions:
const add = (a, b) => a + b;
- Template Literals
const greeting = `Hello, ${name}!`;
Conclusion
And that’s a wrap! We’ve just scratched the surface of the amazing world of CSS and JavaScript. Remember, practice is key, and the web is your canvas. Go out there and create something awesome!
In the next blog post we’ll cover frameworks for CSS and JavaScript, pick one for each and create a Pokemon search application.
Leave a Comment