This is How Your Parents Used to Build Websites
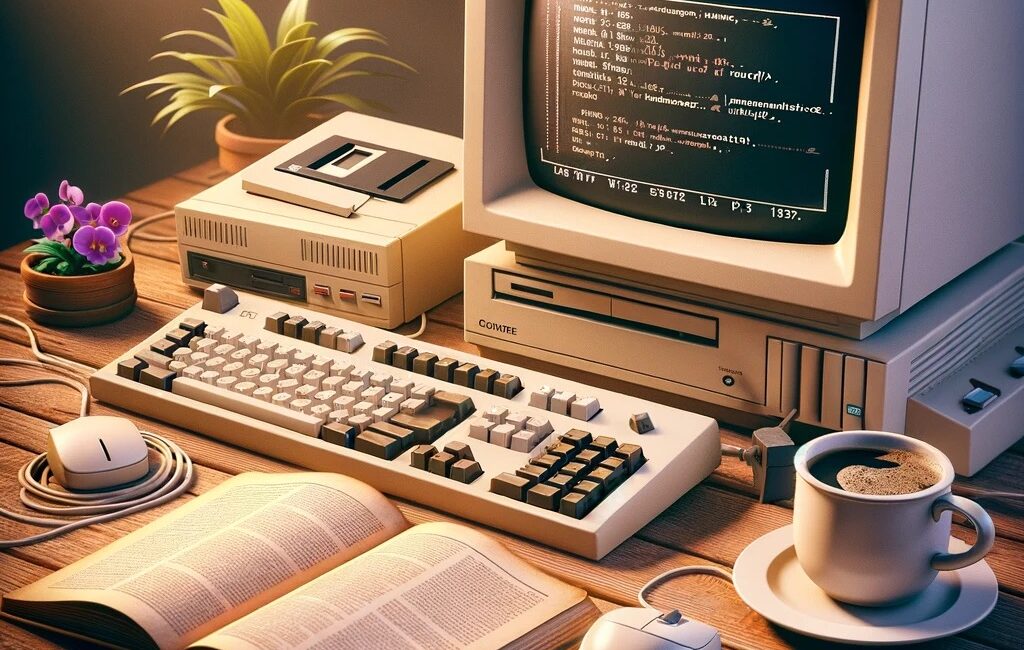
TL;DR
Hey future web wizards! Are you ready to embark on your journey into the vast world of web development? This post is your beginner-friendly guide to HTML. We’ll break down the essentials, infuse some humor, and provide plenty of examples for you to experiment with. By the end of this, you’ll be well on your way to crafting your first web page. Let’s dive into the world of tags and elements and make them your new best friends!
The intro you just read might sound like a blast from the past – a time when all aspiring web developers had were books and a computer (if they were lucky). Back then, the web was a simpler place, primarily text and links.
But hold on, we’re not just reminiscing about the old days. Even though web development has evolved tremendously (and yes, it’s a lot cooler now), understanding the basics of HTML is still crucial. So, even if you’re planning to leap into more advanced topics (which we’ll cover in the next blog post), this article is worth your time to grasp the fundamentals of HTML tags.
In this blog post we’ll cover HTML basics. In the next one we’ll touch on CSS and JavaScript.
Setting the Scene
Picture this: It’s your first day as an aspiring web developer, buzzing with ideas and ready to make your mark on the internet. But there’s a key skill you need to master first: HTML. Don’t fret – it’s far from daunting. HTML is the skeleton of all websites, and together, we’re going to unravel its mysteries.
Why HTML, Anyway?
HTML stands for HyperText Markup Language. It’s the skeleton of all web pages. Think of it as the foundation of a house – without it, you can’t build anything stable. HTML is where every web developer begins their journey. It’s simple, powerful, and the stepping stone to more complex web technologies.
OK, but What’s in a Markup Language?
Markup languages are all about annotating text to give it structure and meaning. HTML does this by using tags to turn plain text into a structured, visually appealing web page. It’s like taking a plain old document and adding magic to make it a web page.
⚠️ And, yes, to settle the debate: No, HTML is not programming. Sorry, not sorry.
Your First HTML Tags
Let’s start with the basics. Every HTML document has some key tags:
<!DOCTYPE html>
: This declaration defines the document type and HTML version<html>
: The root element that wraps the entire content<head>
: This contains meta-information about the document, like its title<body>
: The meat of the page – this is where all your content will go
Creating Your First HTML Page
Open up your favorite text editor (Visual Studio Code, Sublime Text, Vim, … – take your pick) and let’s get coding. Copy the following code into a file and name it index.html
:
<!DOCTYPE html>
<html>
<head>
<title>My First Web Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>Welcome to my first web page.</p>
</body>
</html>
Now open this index.html
file in your favorite browser (Chrome, Safari, Firefox, …). Usually, just doubleclicking the file should make it automatically open in your default browser.
Voila! You’ve just created your first web page that should look like this:
Understanding the HTML Structure
HTML documents are structured as a tree of elements. Each element has an opening tag (<tag>
) and a closing tag (</tag>
). Some elements are self-closing, like <img />
(for inserting images) and <br />
(for inserting a new line). Think of it as a family tree, but for your web content.
Dive into Elements and Attributes
HTML elements can have attributes that provide additional information about the element. For example, <a href="http://www.nikola-breznjak.com/">My site</a>
uses the href
attribute to define the link’s destination.
Text Formatting and Layout
HTML offers a range of elements for formatting text:
<h1>
to<h6>
: Headings from most to least important<p>
: Paragraphs<strong>
and<em>
: Bold and italic text for emphasis<ul>
and<ol>
: Unordered (bullets) and ordered (numbers) lists<li>
: List items<div>
: A generic container for content, great for layout and styling purposes
Adding Images
To add an image, use <img src="image.jpg" alt="Description">
. Change the image.jpg
with an URL of an actual image. For example, edit the index.html file to look like this:
<!DOCTYPE html>
<html>
<head>
<title>My First Web Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>Welcome to my first web page.</p>
<img src="https://i.imgur.com/AoX2x7f.png" alt="VIM for Life">
</body>
</html>
And you should get this:
Adding Links
To create a link, use the a
tag. Example: <a href="http://www.google.com">Link to Google.com</a>
.
Forms and User Input:
Forms are what you should use to get some input from users. Use the <form>
element to create an input area. Inside, use <input>
, <textarea>
, <button>
, and other form elements to gather user input.
For example, here’s the usual login form:
<form action="/submit-your-login-form" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<button type="submit">Login</button>
</form>
If you add that to the index.html
file, you should see this in your browser (when you refresh the page):
Embedding Media
You can embed videos and audio in HTML5 using <video>
and <audio>
tags. Here’s an example for video:
<video width="320" height="240" controls>
<source src="movie.mp4" type="video/mp4">
<source src="movie.ogg" type="video/ogg">
Your browser does not support the video tag.
</video>
and here’s the example for audio:
<audio controls>
<source src="audio.mp3" type="audio/mpeg">
<source src="audio.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
Of course, you would have to have the video and audio formats to try this out. You can Google, or create some of your own. Or, for the sake of quickly testing this out, here’s a link to an MP3 of a crowbar sound from a cult game Half Life.
Building a Simple Personal Portfolio Site with HTML
Let’s enhance our basic HTML skills by creating a simple personal portfolio page. This website will have four main sections: a header, an about me section, a projects section, and a contact section.
Creating the Basic Structure
Start by defining the structure of your page. Open your text editor and create a new file named portfolio.html
. Here’s how you’ll structure it:
<!DOCTYPE html>
<html>
<head>
<title>My Personal Portfolio</title>
</head>
<body>
<header>
<!-- Header content goes here -->
</header>
<section id="about">
<!-- About me content goes here -->
</section>
<section id="projects">
<!-- Projects list goes here -->
</section>
<section id="contact">
<!-- Contact information goes here -->
</section>
</body>
</html>
Crafting the Header
The header will contain your name and a navigation menu.
<header>
<h1>John Doe</h1>
<nav>
<ul>
<li><a href="#about">About Me</a></li>
<li><a href="#projects">Projects</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
The About Me Section
Here, you’ll introduce yourself. Include a brief paragraph and maybe a profile picture.
<section id="about">
<h2>About Me</h2>
<img src="profile.jpg" alt="John Doe" />
<p>Hi, I'm John Doe, a passionate web developer...</p>
</section>
Showcasing Projects
In the projects section, list some of the work you’ve done. Use <ul>
for the list and <li>
for each project.
<section id="projects">
<h2>Projects</h2>
<ul>
<li><a href="http://example.com/project1">Project 1</a></li>
<li><a href="http://example.com/project2">Project 2</a></li>
<!-- Add more projects here -->
</ul>
</section>
The Contact Section
Include your contact information or a form for visitors to reach out to you.
<section id="contact">
<h2>Contact Me</h2>
<p>Email: [email protected]</p>
<!-- You can add a contact form here in future -->
</section>
Adding Some Styling
While CSS is primarily used for styling, you can add inline styles directly in your HTML to change the look of your page. For example:
<style>
body {
font-family: Arial, sans-serif;
}
header, section {
margin-bottom: 20px;
}
nav ul {
list-style-type: none;
}
nav ul li {
display: inline;
margin-right: 10px;
}
</style>
Place this <style>
tag within the <head>
section of your HTML.
Test and Iterate
This is how the full portfolio.html
file should look like:
<!DOCTYPE html>
<html>
<head>
<title>My Personal Portfolio</title>
<style>
body {
font-family: Arial, sans-serif;
}
header, section {
margin-bottom: 20px;
}
nav ul {
list-style-type: none;
}
nav ul li {
display: inline;
margin-right: 10px;
}
</style>
</head>
<body>
<header>
<h1>John Doe</h1>
<nav>
<ul>
<li><a href="#about">About Me</a></li>
<li><a href="#projects">Projects</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<section id="about">
<h2>About Me</h2>
<img src="profile.jpg" alt="John Doe" />
<p>Hi, I'm John Doe, a passionate web developer...</p>
</section>
<section id="projects">
<h2>Projects</h2>
<ul>
<li><a href="http://example.com/project1">Project 1</a></li>
<li><a href="http://example.com/project2">Project 2</a></li>
<!-- Add more projects here -->
</ul>
</section>
<section id="contact">
<h2>Contact Me</h2>
<p>Email: [email protected]</p>
<!-- You can add a contact form here in future -->
</section>
</body>
</html>
Open it up in a browser to see your work. It won’t look like a polished website yet, but you’ll see all your content structured according to your HTML.
Conclusion
Congratulations! You’ve just built a simple yet functional personal portfolio site using HTML. This is just the beginning of your journey into web development. As you learn more about HTML, CSS, and JavaScript, you’ll be able to enhance your site with better styling and functionality. Keep experimenting, keep learning, and most importantly, have fun with it!
In the next blog post we’ll cover CSS and JavaScript.
Leave a Comment