Making AJAX calls using jQuery
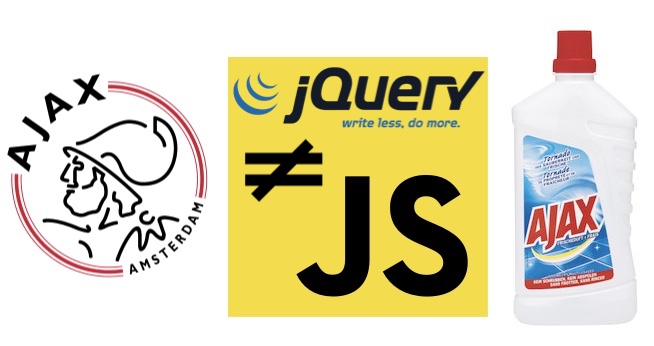
TL;DR
In this post we’ll do everything (and a bit more ?) we did in the first post, but with jQuery.
Why is jQuery better?
I prepared this demo page so that you can test.
You’ll remember from the last post that in order to make an AJAX call in pure JavaScript, you have to do something like this:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4){
document.getElementById('result').innerHTML = xhr.responseText;
}
};
xhr.open('GET', 'http://nikola-breznjak.com/_testings/ajax/test1.php?ime=Nikola');
xhr.send();
Go ahead and run that code on the demo page in the browser’s dev tools Console (consult the last post if you’re stuck).
Now, to do the very same thing with jQuery, all you have to do is this:
$('#result').load('http://nikola-breznjak.com/_testings/ajax/test1.php?ime=Nikola');
Go ahead and try it in the Console. Change the ime
parameter, and you’ll see that the text on the page will change to Hello [name]
, where [name] will be the parameter you entered.
⚠️ I won’t go into the basics of using jQuery, as it’s been here for a very long time, and there’s a vast amount of tutorials written about it. Start with the official docs if you want to learn more.
The important thing to note here is that .load
must be chained to a so-called jQuery selection, as mentioned in the .load() documentation. We loaded the data from the server and placed the returned text into the element with an id result
.
Shorthand methods
By looking at the docs, you can see that there are more of these so-called shorthand methods for making AJAX calls, all of which could be done with the most versatile ajax function.
The example from before could be done using the get shorthand method:
var link = 'http://nikola-breznjak.com/_testings/ajax/test1.php';
var data = {ime:"Nikola"};
var callback = function(result) {
$('#result').html(result);
}
$.get(link, data, callback);
If you don’t want to pass any parameters, it gets even simpler:
var link = 'http://nikola-breznjak.com/_testings/ajax/test1.php';
var callback = function(result) {
$('#result').html(result);
}
$.get(link, callback);
Rewriting the mini project
I prepared the same demo page as in the previous post, but with jQuery already included so you can test this by just pasting the code below in the Console. I encourage you first to try it yourself and then compare your solution with mine below.
var link = 'http://nikola-breznjak.com/_testings/ajax/test2.php';
$.getJSON(link, function(result){
var oglasiHTML = '';
$.each(result, function(index, oglas){
var klasaCijene = '';
if (oglas.cijena < 100){
klasaCijene = 'is-success';
}
else if (oglas.cijena >= 100 && oglas.cijena < 300){
klasaCijene = 'is-info';
}
else if (oglas.cijena >= 300){
klasaCijene = 'is-danger';
}
oglasiHTML += `
<div class="columns">
<div class="column is-one-quarter">
<span class="tag ${klasaCijene}">${oglas.cijena}</span>
</div>
<div class="column">${oglas.tekst}</div>
</div>
`;
});
$('#oglasi').html(oglasiHTML);
});
There are a few things that I’d like to point out here:
- I used the getJSON function to call my API located on the URL that’s saved in the
link
variable. This is because I know that this API returns a JSON response (you can check by opening that link in the browser). - I used the jQuery each function to iterate over the
result
. - I used the template literals to construct the final
oglasiHTML
in a much cleaner way than we did that in the previous post with using concatenation. - The core logic remained the same as in the first post, with the exception of not having to use the
for
loop andindexes
to access each element of the array.
I want more examples
Say you have this demo page, and you need to make the newsletter signup form use AJAX instead of going to a new page when you click the Subscribe
button. Right now the API (located at http://nikola-breznjak.com/_testings/ajax/email.php) doesn’t actually process what you send to it, but try to send data with the request as well for practice.
How would you do it? Where would you start?
Well, here are a few search terms you could Google:
- `form submit jquery’
post data
Again, here’s my solution, but I encourage you to try it yourself first.
$('form').submit(function(ev){
ev.preventDefault();
var url = $(this).attr('action');
var formData = $(this).serialize();
$.post(url, formData, function(response){
$('#newsletter').html('Thank you for subscribing, please check your email.');
});
});
There are a few things that I’d like to point out here:
- I was able to use the
form
selector without any id because it’s the only form on the page. If there were more forms on the page, I’d have to distinguish them by using an id (which is always a good practice, even if it’s just one form) - I used jQuery’s submit function to set an event handler that will be executed when the form will be submitted (
Subscribe
button clicked, or ENTER pressed while in one of the fields) - I used the
ev.preventDefault()
to prevent the form from “doing its thing” and loading the ’email.php’ page, as its default behavior - I used
$(this).attr('action')
to extract the API URL from the form definition it the HTML (feel free to check it out in the element inspector) - I used
$(this).serialize()
to encode a set of form elements as a string which I then passed to the jQuery post shorthand function. This function sends a request to the server using the POST method, which (as we learned in the previous post) is the preferred way of sending some sensitive data that needs to be handled on the server - I used the html function on a
div
with the idnewsletter
to set its new content
Can I have one more, please?
Sure, but promise you’ll do it yourself first!
I do ?
OK then, let’s do this ?
Here’s a new demo page that you’ll use for this example.
⚠️ I’m using Bulma to make the site look pretty. As their website says:
‘Bulma is a free, open source CSS framework based on Flexbox and used by more than 150,000 developers.’
I’ll just say it’s really great and easy to learn, so make sure you check it out.
In this challenge, you have to create a gif search application using the Giphy Search API. As the docs state, you’ll need to get the API key by creating an app. Once you do that, you’ll be able to search their API like, for example, this: http://api.giphy.com/v1/gifs/search?q=funny+cat&api_key=dc6zaTOxFJmzC
. You can use this API key, but be sure to create your own if this one gets banned for too many requests ?
Once you fetch the gifs (or still images if you so choose), show them in the div with a class result
(yes, class, not id ?).
Here’s the part where you roll up your sleeves, create it, feel proud of yourself ?, and then come here to check how I did it.
$('form').submit(function(ev){
ev.preventDefault();
var searchTerm = $('input').val();
var apiLink = "http://api.giphy.com/v1/gifs/search?api_key=dc6zaTOxFJmzC&q=" + searchTerm;
var output = '';
$.getJSON(apiLink, function(images){
$.each(images.data, function(i, im){
output += `<img src="${im.images.downsized.url}"/>`;
});
$('.result').html(output);
});
});
The only new, and potentially tricky, part here is the traversal of the JSON object that you get back from the API, but with just a bit training, you’ll get good at it.
If you open up this URL in your browser, you will see this
The returned JSON object consists of three properties:
data
pagination
meta
The data
is an array of objects, and in one of its properties it has an array of images
. In my example, I choose to display the downsized
gif by accessing its url
property.
Here’s a short gif of this in action:
Conclusion
That’s all for this blog post. I hope it was useful and that you’ve seen how much easier it is to make AJAX requests with jQuery vs. plain JavaScript. I also hope you liked the demo exercises ?
In the next blog post, we’ll see how to do this with the modern fetch API.
Leave a Comment