Simple caching with Cache_Lite without having to install it through PEAR
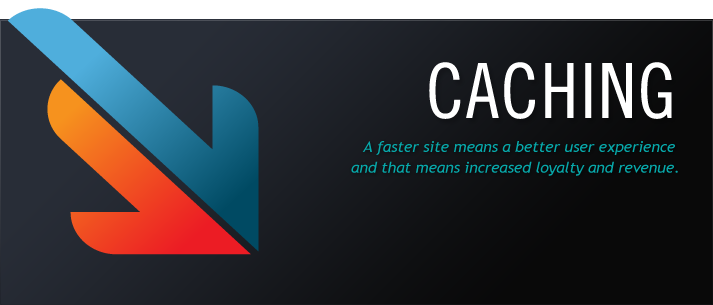
Sooner or later everyone has to make use of some kind of caching of their content. Below is the simplest possible code demonstration on how to implement caching with Cache_Lite (download Cache_Lite standalone PHP class file which you can just include to your project without having to install it through PEAR):
require_once('Cache_Lite.php'); $cache = new Cache_Lite( array( 'cacheDir' => "cache/", 'lifeTime' => 20 //seconds ) ); $req = "file.txt"; if($data = $cache->get($req)) { echo "Result from cache: " . $data; } else { $data = file_get_contents($req); // this is usually a DB call! $cache->save($data); echo "Result not from cache: " . $data; }
So, first you require the class file, make a new instance by setting a caching directory and cache lifetime. For demonstration purposes I’m outputing the contents of file.txt but of course in a real use case scenario that would be some result of a database query or sth like that.
Simply, to get the data from cache (if it exists) you have to call the get() function on the Cache_Lite object, and to save it, well, use save() function and you’re all done.
Leave a Comment