How to create the simplest TODO application with Ionic 3
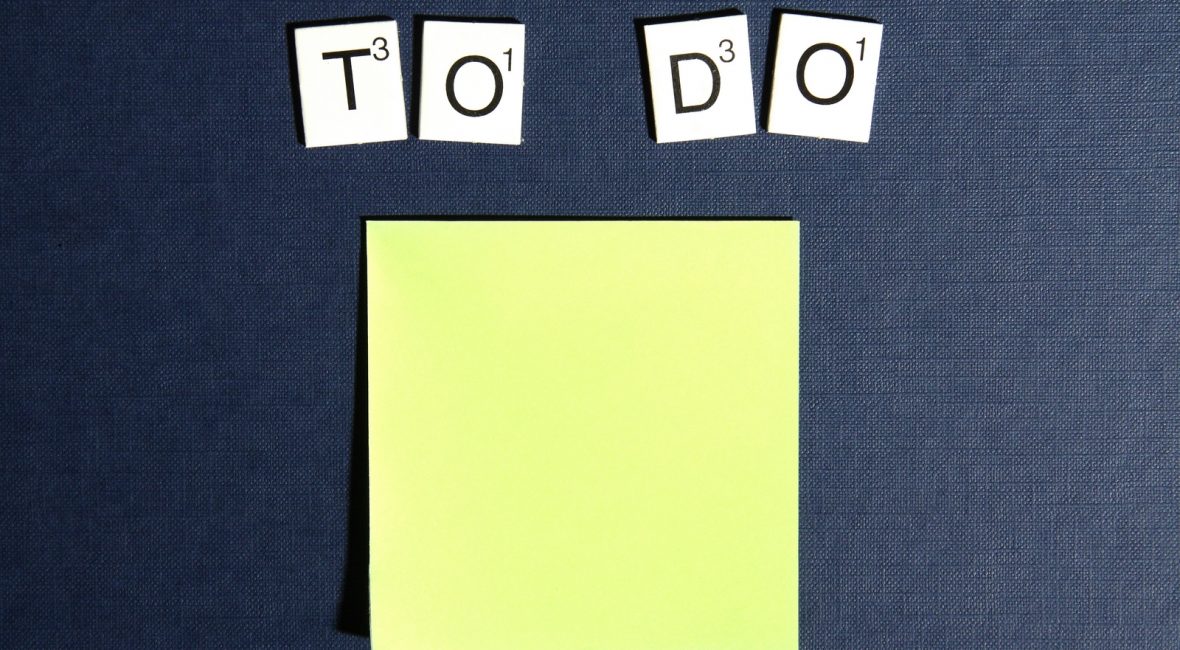
TL;DR
This is the simplest example which shows how to create a simple TODO application with Ionic 3 in just a few lines of code.
Quickstart
To see it in action:
- Clone the finished project from Github
- Make sure you’ve got the newest Ionic CLI (see this post for more instructions)
- Run
ionic serve
- The app in action (after adding few items and swiping to delete one):
Step by step on how to create this yourself from scratch
Create a new blank Ionic project with ionic start Ionic3ServerSendTest blank
Template
Copy the following code to src/pages/home/home.html
file:
Here we have three parts:
- an input (
ion-input
) field where we enter the text for our TODO. We’ve put a ngModel of nametodo
on it – we will be able to reference this in the code by this same variable name. - a button (
ion-button
) which when clicked calls the function calledadd
, which we’ll define in oursrc/pages/home/home.ts
file shown below. - a list (
ion-list
) with multiple items (ion-item
) in it. Also, we’ve made use of theion-item-sliding
(learn more about it from the docs here). We are repeating theion-item-sliding
component as many times as we have items in thetodos
array (you’ll see where we set that in thehome.ts
file, as mentioned above).
Code
To the src/pages/home/home.ts
file copy the following content:
import { Component } from '@angular/core';
import { NavController } from 'ionic-angular';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
todos: string[] = [];
todo: string;
constructor(public navCtrl: NavController) {
}
add() {
this.todos.push(this.todo);
this.todo = "";
}
delete(item) {
var index = this.todos.indexOf(item, 0);
if (index > -1) {
this.todos.splice(index, 1);
}
}
}
The only thing that differs here from the basic blank
template generated code is the todos
and todo
variables which we use for holding the array of todos and a current todo that’s connected to the model in the input box.
The add
function pushes the current todo
that we have in the input box at the time of pressing the Add
button into the todos
array.
The delete
function first finds the index of the item that we want to delete from the list and then, by using the splice function, it deletes the item from the todos
array.
Running the app
Just run ionic serve
or even better yet ionic lab
from the root directory of your Ionic app and you’ll have the app running locally in your browser tab with live reload option turned on.
Conclusion
That’s it. It doesn’t get any easier than this. Sure, this is as simple as it gets; there’s no persistence or anything, that’s for you to play with. Or, well, I may add that in the next tutorial…
‘Till then, have a great one, and a happy 4th of July to all my friends across the big pond 😉
How to create the simplest #TODO application with #Ionic 3 https://t.co/xvjmXVruBH
— Nikola Brežnjak (@HitmanHR) July 4, 2017
Leave a Comment