Converting a JavaScript switch statement into a function lookup
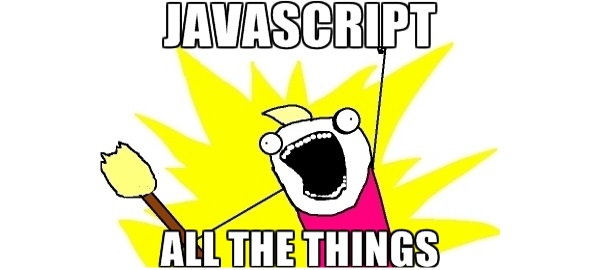
I just read a great post on WebTools Weekly newsletter about how to convert a JavaScript switch statement into a function lookup. Here’s the full content from there, so be sure to check it out if you like it (it’s one newsletter a week, and no – I’m not affiliated with them in any way).
Because of the problems inherent in using JavaScript’s switch statement, many developers and reference guides will recommend avoiding switch constructs and instead using something called amethod lookup (also referred to as a lookup table or even dispatch table). Let’s convert a switchstatement to a method lookup, so we can see how this is done. Here’s our switch:
function doSomething(condition) { switch (condition) { case 'one': return 'one'; break; case 'two': return 'two'; break; case 'three': return 'three'; break; default: return 'default'; break; } }
All of this is inside a doSomething() function, with a condition passed in. The possible values are ‘cased’, to define the return value. This is cleaner than a messy if-else construct, but can we clean it up even more? Here’s basically the same thing in the form of a method lookup:
function doSomething (condition) { var stuff = { 'one': function () { return 'one'; }, 'two': function () { return 'two'; }, 'three': function () { return 'three'; } }; if (typeof stuff[condition] !== 'function') { return 'default'; } return stuff[condition](); }
In the demo, you can see four logs in the console, one for each valid condition and then another one to demonstrate the default condition when the argument doesn’t match one of the methods.
You can see why people like this technique. It’s clean, elegant, and seems a little more sophisticated without being more complex. I’ve only scratched the surface on this, so here are a few good resources to read up more on this subject:
- Don’t Use Switch in Programming JavaScript Applications by Eric Elliot.
- Using Dispatch Tables to Avoid Conditionals in JavaScript by Josh Clanton
i have seen same thing done with if else loop but forgot what should be the last return statement.
example was something like this:
function a(val){
var opt= {
‘car’:return ‘car’,
‘foo’:return ‘foo’
}
return