Intro to OOP in JavaScript
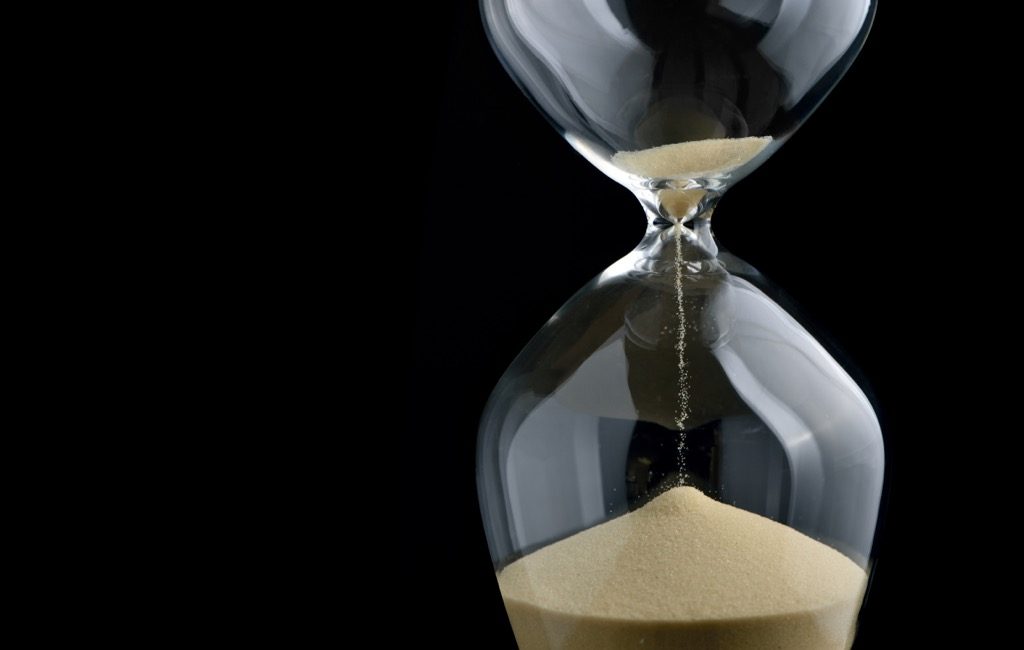
TL;DR
This post will not be a regular step by step post as I usually do it; this will rather be a collection of some notes from a recent lecture I did. However, I hope you still find it useful.
OOP – Object Oriented Programming
- an object has a
state
(represented byproperties
) and abehavior
(represented bymethods
) functions
on an object are calledmethods
- a developer doesn’t need to know how an object’s methods work under the hood to be able to use them (encapsulation)
- a lot of times it makes sense to model real-life objects as objects in the code
⚠️ interesting thing in JavaScript is that
arrays
are actually objectsYou can test it like this:
console.log(typeof []);
It’s an object because it has a property like
length
, and methods likepop
andpush
object literal
const person = {
name: 'Nikola',
age: 33,
walk: function() {
console.log("I'm walking");
}
}
console.log(person.name);
There’s also this thing called ‘bracket notation’:
person['name']
person['walk']();
Here’s how you would output all of the object’s properties with jQuery:
$.each(person, function(i,o){
console.log(i, o);
});
this
in JavaScript
- normal function calls
function f(){
console.log(this); // Window object
}
f();
- object methods
var obj = {
a: "test",
b: "test 2",
log: function() {
console.log(this.a);
}
}
obj.log();
- constructed object methods
var Person = function(name, surname) {
this.name = name || 'Marko';
this.surname = surname || 'Maric';
this.log = function() {
console.log(this.name + " " + this.surname);
}
}
var pero = new Person('Pero', 'Peric'); // constructor function
pero.log();
JavaScript Class Syntax
Object literals fall short when you have to create multiple of them. Having to type each of them out and then in case of a change, change it in all the places would be unmanageable.
Class
is basically a blueprint for objects
that will be created with this blueprint. It contains some base set of properties
and methods
. The class
syntax is new to JS in ES2015
and it’s actually something called ‘syntactic sugar’. It has the same functionality as the prototype syntax, and it’s actually still using prototypes under the hood.
class Pet {
constructor(animal, age, bread, sound) {
this.animal = animal;
this.age = age;
this.bread = bread;
this.sound = sound;
}
speak() {
console.log(this.sound);
}
}
const djoni = new Pet('dog', 1, 'bulldog', 'vau vau');
console.log(djoni);
getters
class Pet {
constructor(animal, age, bread, sound) {
this.animal = animal;
this.age = age;
this.bread = bread;
this.sound = sound;
}
get activity() {
const today = new Date();
const hour = t.getHours();
if (hour > 8 && hour < 20) {
return 'playing';
}
else {
return 'sleeping';
}
}
speak() {
console.log(this.sound);
}
}
const djoni = new Pet('dog', 1, 'bulldog', 'vau vau');
console.log(djoni.activity); // activity is a so-called 'computed property'.
setters
get owner() {
return this._owner.
}
set owner(owner) { // MUST have exactly one formal parameter!
this._owner = owner; // this is called a 'backing property'
}
djoni.owner = 'Nikola';
Leave a Comment