How to run Node.js server in Ionic mobile app?
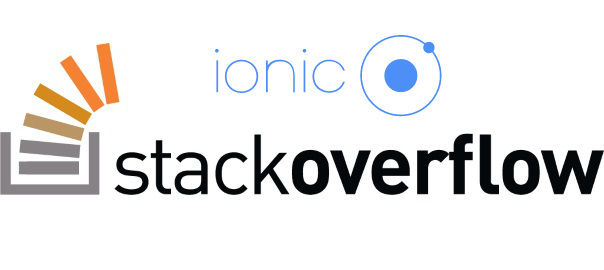
I’m a big fan of Stack Overflow and I tend to contribute regularly (am currently in the top 0.X%). In this category (stackoverflow) of posts, I will be posting my top rated questions and answers. This, btw, is allowed as explained in the meta thread here.
As you may know, I’m really into Ionic framework lately and am helping out on StackOverflow with the knowledge I gained so far with the framework. I’m currently #1 in the top All time answerers list.
I answered this question by user Shubham:
I am making an app using MEAN and ionic framework where nodejs is a middleware to connect to the database(mongoDb). I need to run the nodejs server using node server.js
and the app using ionic serve
. This is my server.js.
var express = require('express'), app = express(), bodyParser = require('body-parser'), mongoose = require('mongoose'), CohortController =require('./www/server/controller/CohortController'); mongoose.connect('mongodb://localhost:27017/persistent'); app.use(bodyParser()); app.get('/api/cohorts',CohortController.list); app.post('/api/cohorts',CohortController.create); app.listen(3000,function(){ console.log('Listening...'); })
Now this is my app.js. I use http://localhost:3000
to get the JSON.
app.controller('CohortController',['$scope','$resource', function($scope,$resource){ var Cohort=$resource('http://localhost:3000/api/cohorts'); Cohort.query(function(results){ $scope.cohorts=results; }); $scope.cohorts=[]; $scope.createCohort= function () { var cohort=new Cohort(); cohort.name=$scope.CohortName; cohort.id=$scope.CohortId; cohort.$save(function(result){ $scope.cohorts.push(result); $scope.CohortName=''; $scope.CohortId=''; }); } }]);
How can I run the node server when I convert it into a mobile application? How the application will use the API?
My answer was:
You will have to have your Node.js app running on a server which you would then access (from your Ionic app) via it’s public IP. So, you wouldn’t use
http://localhost:3000
to get the JSON, instead you would use something likehttp://123.456.789.123:3000
.But, usually, this is not the way you would do it (with the port 3000). What you would additionally do is put (for example) Nginx in front of your Node.js app (see an example here) in order to serve your api from the standard HTTP port (80).
So, basically, you can’t actually “run Node.js server in Ionic app” – the way you do it is run the Node.js app separate from Ionic and expose its functionality via a standardized API (usually these days RESTis what you would want to achieve) which you then “consume” via Ionic’s (well, to be exact, it’s actually Angular’s) $resource module.
Hope this helps clear things up a bit.
How to run #Node.js server in #Ionic mobile app? https://t.co/8SOUYGM3Vf
— Nikola Brežnjak (@HitmanHR) January 12, 2016
Hey I am still a little confused. I am use to having the server/api running in the same folder (.NET) so there is no concern for this. I want to create a node express server for an ionic app. Lets say I want to allow the ionic app running on someones phone to access the node api and one web site (example.com) to have access. It sounds like I need to enable CORS, but I don’t want to open it up to attacks so from reading it sounds like we don’t want to do: ‘Access-Control-Allow-Origin’, ‘*’. If I just wanted the website I would do ‘Access-Control-Allow-Origin’, ‘example.com’. I am lost how to allow calls from phones running the ionic app. Any advice is appreciated. Let me know if I need to clarify anything, new to this stack; coming from .NET world.
Hey Chris, just so I don’t leave this unanswered – I answered on SO: http://stackoverflow.com/questions/34579550/how-to-run-node-js-server-in-ionic-mobile-app/34580053?noredirect=1#comment60002729_34580053
Hi Nikola!
I am Studying programming and for my final project in school, I decide to do something different from the technologies I learn during my classes .Net Java, etc.
I take the risk to learn in little time, the creation of an hybrid app using Ionic Framework and use backend services as MEAN stack. Now I am trying to learn as fastest I can how to implement my app.
I read some post you wrote and I feel you really know what you say, so I write to you in order to ask for some guidance if you have time.
I am totally new to ionic, and mean. I manage to install Ionic in ubuntu and create a blank app and emulate it in android and works, but now I have to test, how to connect my ionic app, to mean, in order to create data in my mongo db, retrive data and display in Ionic. The app i am trying to make is about training. Well I hope not to overkill you with my post, my english isnt my native language, thanks for any guidance you could give me.
Sincerely George.
Hi George,
First of all, I congratulate you on your guts to go out of your comfort zone and do something else. That’s always a good trait to have, so just stick with it and you’ll do good in life!
So, since I really like people who take initiative I’ll help you as much as I can.
To connect Ionic and MEAN you will have to create a REST endpoint in your MEAN stack and you’ll then be able to consume it with Angular via its $resource service.
Few links that may help: http://stackoverflow.com/questions/34579550/how-to-run-node-js-server-in-ionic-mobile-app/34580053, https://scotch.io/tutorials/build-a-restful-api-using-node-and-express-4.
Also, you probably know this, you can download my book for MEAN and Ionic for free (if you can’t find the links on my site, ping me and I’ll paste them here).
So, to summarize, you first need to create “something” (this is usually REST these day) on your backend that will then serve data back to any client (in our case this will be an Ionic app and access will be made via Angular’s $http or $resource services).
Your English is great, btw, and if you have any more specific questions, don’t hesitate to ping.
Good luck!
Hello Nikola,
I am currently in the exact same situation as George, final year project due in three weeks; would you mind pasting the link to your book please?
Best regards.
Sure, no probs, it’s here: https://leanpub.com/ionic-framework
Hi Nikola,
I’m in a similar situation wanting to create an app with MEAN + Ionic rather fast. I downloaded your book for Ionic but from your comments I thought there was a book for MEAN stack too. If that is the case I’d really appreciate if you could paste the link. Thank you!
Hi Rafael,
Yes, the MEAN book is here: https://leanpub.com/meantodo
Hi Nikola,
Thanks for your insightful post. I landed here searching for a question yet unanswered, and seeing your notorious contributions, i would like to have your insight.
So, let’s pretend I want to do a Ionic app similar to AirDroid. As in, create a hotspot, and let other devices connect to myself and serve content. In short, a minimal webserver (off the internet). After checking the cordova-hotspot plugin, I am not yet sure how to implement such project.
All that being said, two questions:
-Is it possible?
-If so, could you give me some brief guidelines?
Thank you for your attention