How to build a 2D Space shooter with efficiency calculation in Unity3D
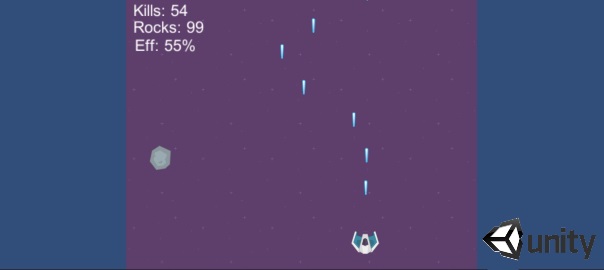
In this blog post I’m going to show you how to build a 2D Space shooter with efficiency calculation in Unity3D.
I followed these two blog posts: post #1 and post #2, and updated it for the use with Unity 4.5 and added the counters for number of bullets fired and number of rocks generated and showed the precision efficiency.
You can try my version, or you can download the project on GitHub. Following are the steps on how to make it your self:
- Start a new 2D project in Unity3D
- Create folders Scripts, Scenes, Prefabs, Textures
- Put all the texture assets to Textures folder
- Save the (ctrl +s) scene to Scenes folder with some name
- Drag background to Hierarchy
- Drag ship to Hierarchy
- set y to -4
- add Rigidbody2D
- check IsKinematic (the gravity doesn’t affect it)
- Add script to ship:
#pragma strict // A variable that will contain our bullet prefab public var bullet : GameObject; public var brzina: int; var score : int; function Start(){ score = 0; } function Update() { // Move the spaceship horizontally rigidbody2D.velocity.x = Input.GetAxis("Horizontal") * 10; rigidbody2D.velocity.y = Input.GetAxis("Vertical") * 10; //add support for mobile phone tilting transform.Translate(Input.acceleration.x*Time.deltaTime*20, 0, 0); if (Input.GetKeyDown("space") || Input.GetMouseButtonDown(0)) { Instantiate(bullet, transform.position, Quaternion.identity); } }
- Add bullet to Hierarchy
- add Physics2D -> Rigidbody 2D
- add script:
public var speed : int = 6; // Gets called once when the bullet is created function Start () { // Set the Y velocity to make the bullet move upward rigidbody2D.velocity.y = speed; } // Gets called when the object goes out of the screen function OnBecameInvisible() { // Destroy the bullet Destroy(gameObject); }
- add bullet to Prefabs folder and delete it from the Hierarchy
- drag the bullet from Prefabs folder to the bullet variable in Inspector when the spaceship is selected
- Add an enemy (from Textures) to the Hierarchy
- add Rigidbody 2D
- set IsKinematic
- add script:
// Public variable that contains the speed of the enemy public var speed : int = -5; // Function called when the enemy is created function Start () { // Add a vertical speed to the enemy rigidbody2D.velocity.y = speed; // Make the enemy rotate on itself rigidbody2D.angularVelocity = Random.Range(-200, 200); // Destroy the enemy in 3 seconds, // when it is no longer visible on the screen Destroy(gameObject, 3); } function OnTriggerEnter2D(obj : Collider2D) { var name = obj.gameObject.name; // If it collided with a bullet if (name == "bullet(Clone)") { // And destroy the bullet Destroy(obj.gameObject); handleDestroy(gameObject); } // If it collided with the spaceship if (name == "spaceship") { handleDestroy(gameObject); } } function handleDestroy(gameObject: GameObject){ gameObject.Find("ScoreText").SendMessage("Hit"); Destroy(gameObject); }
- Add enemy from Hierarchy to Prefabs folder and delete it from Hierarchy
- Add spawn object to Hierarchy
- position it above the background
- add script:
-
public var enemy : GameObject; // Variable to know how fast we should create new enemies public var spawnTime : float = 1.3; function Start() { // Call the 'addEnemy' function every 'spawnTime' seconds InvokeRepeating("addEnemy", spawnTime, spawnTime); } // New function to spawn an enemy function addEnemy() { // Variables to store the X position of the spawn object // See image below var x1 = transform.position.x - renderer.bounds.size.x/2; var x2 = transform.position.x + renderer.bounds.size.x/2; // Randomly pick a point within the spawn object var spawnPoint = new Vector2(Random.Range(x1, x2), transform.position.y); // Create an enemy at the 'spawnPoint' position Instantiate(enemy, spawnPoint, Quaternion.identity); }
- drag enemy prefab to spawn object
- For the spaceship, the enemy prefab, and the bullet prefab do the following
- add Component -> Physics 2D -> Box Collider 2D
- for enemy Box Collider 2D check IsTrigger and this will give you the OnTriggerEnter2D function
- Create -> UI -> Text (name it ScoreText)
- Canvas -> RenederMode -> World space, no camera
- Rect transform – 450×340 (WxH)
- Add -> Script -> ScoreScript.js:
import UnityEngine.UI.Text; var Counter : int; var text : UnityEngine.UI.Text; function Start () { text = GetComponent(UnityEngine.UI.Text); Counter = 0; } function Update () { text.text = "Kills: "+Counter; } function Hit () { Counter++; }
- Create -> UI -> Text (name it RocksText)
- Add -> Script -> RocksScript.js:
import UnityEngine.UI.Text; var Counter : int; private var text : UnityEngine.UI.Text; function Start () { text = GetComponent(UnityEngine.UI.Text); Counter = 0; } function Update () { text.text = "Rocks: "+Counter; } function RockAdd () { Counter++; }
- Add -> Script -> RocksScript.js:
- Create -> UI -> Text (name it EffText)
- Add -> Script -> EffScript.js:
import UnityEngine.UI.Text; private var Kills : int; private var Rocks : int; private var Eff : float; private var text : UnityEngine.UI.Text; function Start () { text = GetComponent(UnityEngine.UI.Text); Eff = 0; Kills = 0; Rocks = 0; } function Update () { Kills = gameObject.Find("ScoreText").GetComponent(ScoreScript).Counter; Rocks = gameObject.Find("RocksText").GetComponent(RocksScript).Counter; if (Kills == 0 || Rocks == 0) Eff = 0; else Eff = Kills * 1.0f / Rocks * 100; text.text = "Eff: " + Eff.ToString("F0") + "%"; }
- Add -> Script -> EffScript.js:
Leave a Comment