Code Complete 2 – Steve McConnell – Fundemental Data Types
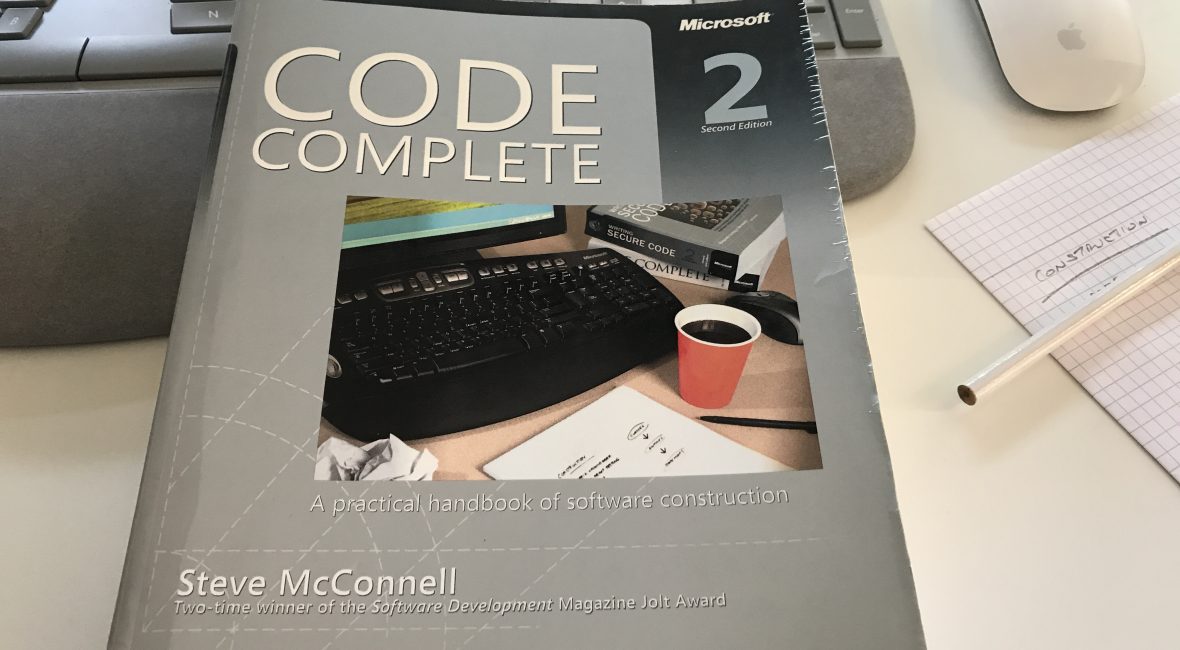
I just love Steve McConnell’s classic book Code Complete 2, and I recommend it to everyone in the Software ‘world’ who’s willing to progress and sharpen his skills.
Other blog posts in this series:
- Part 1 (Chapters 1 – 4): Laying the Foundation
- Chapter 5: Design in Construction
- Chapter 6: Working Classes
- Chapter 7: High-Quality Routines
- Chapter 8: Defensive programming
- Chapter 9: Pseudocode Programming Process
- Chapter 10: General Issues in Using Variables
- Chapter 11: General Issues in Using Variables
The fundemental data types are the basic building blocks for all other data types.
Numbers in General
Avoid “magic numbers” – if you can program in a language that supports named constants, use them instead. A good rule of thumb is that the only literals that should occur in the body of a program are 0 and 1.
Integers
Bear these considerations in mind when using integers:
+ Check for integer division – when you’re using integers, 7/10 does not equal 0.7. It ussually equals 0
+ Check for integer overflow – you need to be aware of the largest possible integer. The larges possible unsigned integer is often 232.
+ Check for overflow in intermediate results
+ ( 1000000 * 1000000 ) / 1000000 = -727
– That’s because the intermediate result is too large for the integer data type
Floating-Point Numbers
Avoid additions and subtractions on numbers that have greatly different magnitudes, 1,000,000.00 + 0.1 probably produces an answer of 1,000,000.00 because 32 bits don’t give you enough significant digits to encompass the range between 1,000,000 and 0.1.
Change to binary coded decimal (BCD) variables. This is a roll-your-own approach to BCD variables. This is particlarly valuable if the variables you’re using represent dollars and cents or other quantities that must balance precisely.
Boolean Variables
Use boolean variables to document your program. Insted of merely testing a boolean expression, you can assign the expression to a variable that makes the implication of the test unmistakable.
Enumerated Types
An enumerated type is a type of data that allows each member of a class of objects to be described in English. You can use enumerated types for readability. Instead of writing statements like if chosenColor == 1
you can write more readable expressions like if chosenColor == Color_Red
.
Anytime you see a numeric literal, ask whether it makes sense to replace it with an enumerated type
If language doesn’t have enumerated types, you can simulate them with global variables or classes.
Class Country {
private Country {}
public static final Country China = new Country();
public static final Country France = new Country();
public static final Country England = new Country();
}
Named Constants
A named constant is like a variable except that you can’t change the constant’s value once you’ve assigned it. Named constanst enable you to refer to fixed quantities, such as the maximum number of employees, by name rather than a number – MAXIMUM_EMPLOYEES rather than, 100, for instance.
Arrays
Arrays are the simplest and most common type of structured data. An array contains a group of items that are all of the same type and that are directly accessed through the use of an array index.
Make sure that array indexes are within the bounds of the array. The most common problem arises when a program tries to access an array element that’s out of bounds.
Creating Your Own Types (Type Aliasing)
Programmer-defined data types are one of the most powerful capabilities a language can give you to clarify your understanding of a program. Here’s how you’d set up the type definition in C++:
typedef float Coordinate;
This type definition declares a new type, Coordinate
, that’s functionally the same as the type float
. To use the new type, you declare variables with it just as you would with a predefined type such as float
.
Coordinate latitude;
Coordinate longitude;
Coordinate elevation;
Here are several reasons to create your own types:
+ To make modifications easier
+ To avoid excessive information distribution
+ To make up for language weakness – C doesn’t have a boolean type, so you can compensate by creating the type yourself: typedef int Boolean
Leave a Comment