Code Complete 2 – Steve McConnell – Design in Construction
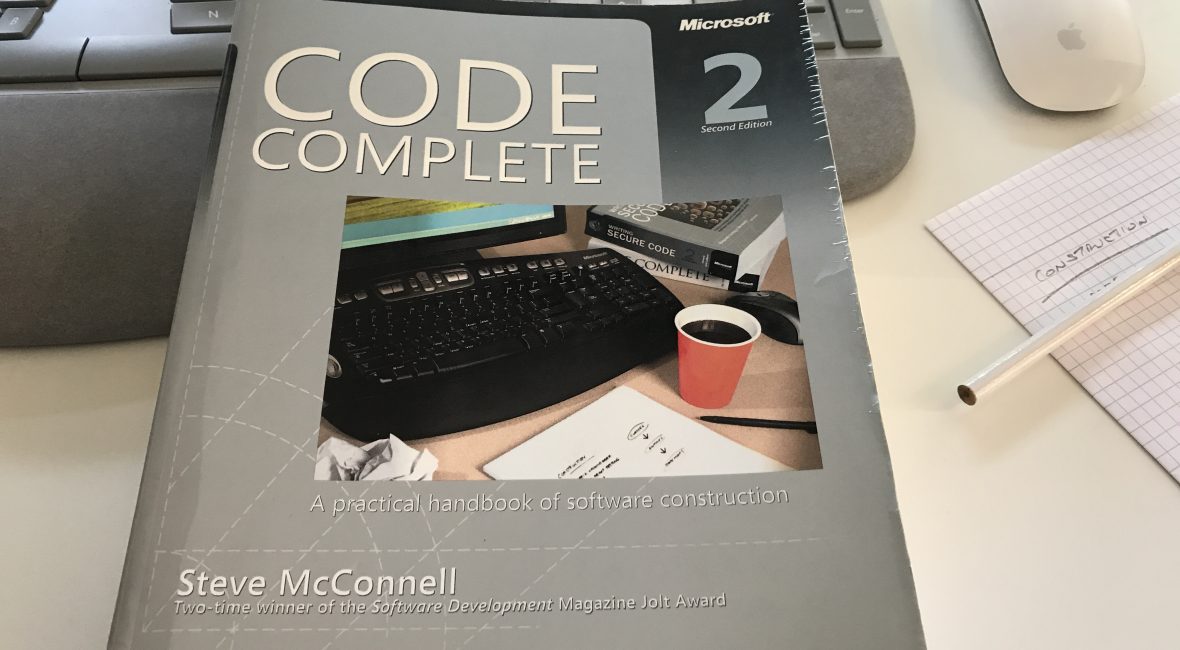
I just love Steve McConnell’s classic book Code Complete 2, and I recommend it to everyone in the Software ‘world’ who’s willing to progress and sharpen his skills.
My notes from Part 1: Laying the Foundation are here.
The second part of the book covers the following chapters:
- Design in Construction
- Working Classes
- High-Quality Routines
- Defensive Programming
- The Pseudocode Programming Process
In this post I’ll share my notes from the:
Chapter 5: Design in Construction
The Wicked problem is the one that could be defined only by solving it or solving a part of it.
An example is Tacoma Narrows bridge. Only by building the bridge (solving the problem) could they learn about the additional consideration in the problem that allowed them to build another bridge that still stands.
According to Fred Brook, there are essential and accidental types of problems.
Managing complexity is the true primary technical goal in software design.
Internal design characteristics:
- minimal complexity
- high fan-in
- ease of maintenace
- low-to-medium fan-out
- minimal connectedness
- portability
- extensibility
- leanness
- reusability
A good general rule is that a system-level diagram should be acyclic. In other words, a program shouldn’t contain any circular relationship in which Class A uses Class B, Class B uses Class C, and Class C uses Class A.
Steps in designing with objects
- Identify the objects and their attributes
- Computer programs are usually based on real-world entities
- Determine what can be done to each object
- A variety of operations can be performed on each object
- Determine what each object can do to the other objects
- This step is just what it sounds like. The two generic things can do to each other are containment and inheritance
- Determine the parts of each object that will be visible to other objects
- One of the key design decisions is identifying the parts of an object that should be made public and those that should be kept private
- Define each object’s public interface
- Define the formal, syntactic, programing-language-level interfaces to each object. The data and methods the object exposes to every other object are called “public interface.”
Heuristics in terms of Software’s Primary Technical Imperative: Managing Complexity.
Summary of Design Heuristics
- Find Real-World Objects
- Form Consistent Abstractions
- Encapsulate Implementation Details
- Inherit When Possible
- Hide Secrets (Information Hiding)
Information hiding is useful at all levels of design, from the use of named constants instead of literals to the creation of data types, to class design, routine design, and subsystem design.
A good class interface is like the tip of an iceberg, leaving most of the class unexposed
Ask yourself what needs to be hidden in this class?
Loose Coupling describes how tightly a class or routine is related to other classes or routines. The goal is to create classes and routines with small, direct, visible, and flexible relations to other classes and routines.
In Software, make the connections among modules as simple as possible.
Life is one big fat program which has a ton of options, but only one nonoptional parameter
The key note is that Classes and routines are first and foremost intellectual tools for reducing complexity. If they’re not making your job simpler, they’re not doing their jobs.
Design patterns
Patterns provide the cores of ready-made solutions that can be used to solve many of software’s most common problems.
- Abstract Factory
- Supports creation of sets of related objects by specifying the kind of set but not the kind of each specific
- Adapter
- Converts the interface of a class to a different interface
- Bridge
- Builds an interface and an implementation in such a way that either can vary without the other
- Composite
- Consists of an object that contains additional objects of its own type so that client code can interact with top-level object and not concern itself with all the detailed
- Decorator
- Attaches responsibilities to an object dynamically, without creating specific subclasses for each possible configuration of
- Facade
- Provides a consistent interface to code that wouldn’t otherwise offer consistent
- Factory Method
- Instantiates classes derived from a specific base class without needing to keep track of the individual derived classes anywhere but the Factory
- Iterator
- A server object that provides access to each element in a set sequentially
- Observer
- Keeps multiple objects in synch with each other by making a third the object responsible for notifying the set of objects about changes
- Singleton
- Provides global access to a class that has one and only one instance
- Strategy
- Defines a set of algorithms or behaviors that are dynamically interchangeable with each other
- TemplateMethod
- Defines the structure of an algorithm but leaves some of the detailed implementation to subclasses
Cohesion refers to how closely all the routines in a class or all the code in the routine support a central purpose
When in doubt use brute force. ~ Butler Lampson
Understand the problem – devise a plan – carry out a plan, look back to see how you did. ~ Polya George
Design practices
- Iterate
- Divide and Conquer
- Top-down and bottom-up
- Experimental prototyping
- You have to be prepared to throw away the code (trick: name the classes -prototype-NameClass )
Put your code in a drawer. Then, come and take it out a week later, and you will criticize the fool who wrote it!
People who preach software design as a disciplined activity spend considerable energy making us all feel guilty. We can never be structured enough or object-oriented enough to achieve nirvana in this lifetime. We all truck around a kind of original sin from having learned Basic at an impressionable age. But my bet is that most of us are better designers than the purists will ever acknowledge. ~ P.J. Plauger
Key note for design is to iterate, iterate, and iterate again and after that, you will be happy with your design.
Conclusion
Hope you liked this post and that you’ve seen that this book really has a lot to offer, so I urge you to get your copy, read it and apply it!
Until next time, take care and keep on improving ?
My #notes from the #book Code Complete 2, Chapter 5 by ever so slightly magnificent Steve McConnell https://t.co/GPV0rq6Upd
— Nikola Brežnjak (@HitmanHR) August 13, 2017
Leave a Comment