Sharing data between scenes in Unity3D
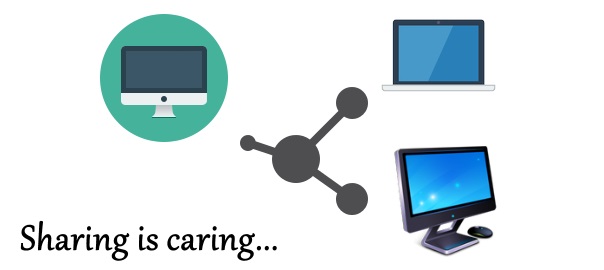
Here’s I’m going to show you steps on how to share your data between scenes in Unity3D
- In the starting scene make a new empty object and name it GameData.
- Add a script named GameController with the following content:
#pragma strict private var data : Array; function Awake () { DontDestroyOnLoad (this); } function Start () { data = new Array(); GetNewResults(); } function GetNewResults(){ var www : WWW = new WWW ("http://localhost/check"); yield www;// Wait for download to complete var dataJson = JSON.Parse(www.data); var novi = dataJson["data"].Value; data = dataJson["someMoreData"]; if (novi == "true"){ Application.LoadLevel(Application.loadedLevel + 1); } else{ Debug.Log("false"); } } public function getData(){ return data; }
The most important part is DontDestroyOnLoad(this) which does not destroy this object once a new scene is loaded.
- Then, in another scene, in some script do the following to fetch the data:
var data = GameObject.Find("GameData").GetComponent(GameController).getData();
Leave a Comment