Wait for images to load and then execute all other code
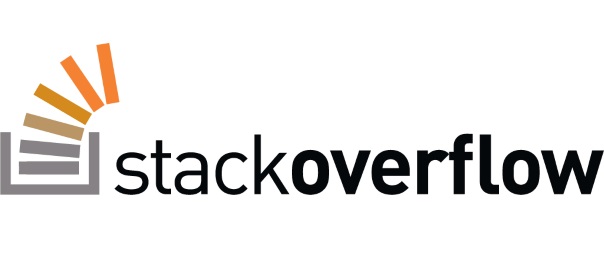
I’m a big fan of Stack Overflow and I tend to contribute regularly (am currently in the top 0.X%). In this category (stackoverflow) of posts I will will be posting my top rated questions and answers. This, btw, is allowed as explained in the meta thread here.
So, my question was as follows:
What I want to do is: wait until all images are preloaded and only then execute all other javascript code. As far as I’m concerned it can (but not a must) have a “loading…” message.
The fact is that I have a pretty big image in the body background and 2 other images which are also bigger, and so I would like to preload them so that then they would show instantly and would not have that ugly “loading” image effect.
This is what I’m using now but it’s not good:
$(document).ready(function() { preloadImages(); ...some other code... function preloadImages(){ imagePreloader([ 'images/1.png', 'images/2.jpg', 'images/3.png', ]); } function imagePreloader(arrayOfImages) { $(arrayOfImages).each(function(){ (new Image()).src = this; }); } }
I don’t know, maybe I should call this preloader somewhere out of the .ready()? or something like that? Btw, yes, I also read this post and I don’t know why but .ready() works faster for me.
The answer, by user Kevin Ennis, was this:
Instead of trying to preload, you could just execute your script on…
window.onload =function(){..}
That doesn’t fire until all images have been loaded.
My own solution was this:
Ok, so finally I got this thing to work. My problem? I was setting the waiting div wrong. This is my code now: I have the loading div which I show above everything and then when all images load (using $(window).load(function(){…}); as suggested I, hide that div.
<divid="loading"><divid="waiting"><imgclass="loadingGif"src="images/loading.gif"></div></div> #loading { background-size: 100%; background-color:#000; width: 100%; height: 100%; margin: 0px; padding: 0px; z-index:999; } #waiting { margin-left: auto; margin-right: auto; position:absolute; top: 39%; left: 27.81%; width: 45%; height: 150px; background-color: #FFF; border: 12px solid #FF771C; text-align: center; }
And my jQuery code is this:
$(window).load(function(){ $('#loading').addClass('hidden');...}
Additionally, user Alex had a great answer too:
I have a plugin named waitForImages that lets you attach a callback when descendent images have loaded.
In your case, if you wanted to wait for all assets to download,
$(window).load()
may be fine. But you could do it a bit cooler with my plugin 🙂var loading = $('#loading'); $('body').waitForImages(function(){ loading.addClass('hidden');},function(loaded, total){ loading.html(loaded +' of '+ total);});
Leave a Comment