Pluralsight WebGL and Three.js fundamentals course notes
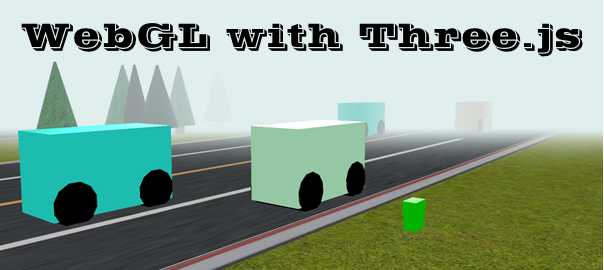
Recently I bought a years worth subscription over at Pluralsight (here’s my portfolio of passed courses), and here I’ll be posting my notes from the courses that I attended. Please note that this is not meant to be as a sort of tutorial, just as the name says – notes for myself :). However, one could get some useful content from these posts as I’m noting things that catch my attention.
Here are my notes from the WebGL and Three.js fundamentals course. I rated the course 4/5 and all in all it’s a good introduction to WebGL with Three.js.
//rant: I passed the exam with the whopping 100%, and actually I feel that since Pluralsight is very famous and all that, that they could improve on the quality (it’s just too easy and does not go into detail) of these certification tests.
var scene = new THREE.Scene(); //acts like a container of all items
var renderer = new THREE.WebGLRenderer(); //how our content will be displayed on the page (WebGL, Canvas or SVG renderers)
It uses a Cartesian cordinate system (x,y,z). If you don’t specify the position- it will be positioned at (0,0,0).
It has a perspective and ortographic camera:
camera = new THREE.PerspectiveCamera( 35, =>fov window.innerWidth / window.innerHeight, 1, => near 1000 => far planes );
You can use your dev tools console (Chrome) or Firebug (Firefox) and access items if you properly export the objects in the code (return {scene: scene}); code snippet in the listing below):
//app.js file var example = (function(){ "use strict"; var scene=new THREE.Scene(), renderer = window.WebGLRenderingContext ? new THREE.WebGLRenderer() : new THREE.CanvasRenderer(), light= new THREE.AmbientLight(0xffffff), camera, box; function initScene(){ renderer.setSize( window.innerWidth, window.innerHeight ); document.getElementById("webgl-container").appendChild(renderer.domElement); scene.add(light); camera = new THREE.PerspectiveCamera( 35, window.innerWidth / window.innerHeight, 1, 1000 ); camera.position.z= 100; scene.add( camera ); box = new THREE.Mesh( new THREE.BoxGeometry(20,20,20), new THREE.MeshBasicMaterial({color: 0x00FF00}) ); box.name="box"; scene.add(box); render(); } function render(){ box.rotation.y +=0.01; renderer.render(scene, camera); requestAnimationFrame(render); } window.onload = initScene; return { scene: scene } })();
//HTML file <!DOCTYPE html> <html> <head> <title>WebGL with three.js Fundamentals</title> </head> <body> <div id="webgl-container"></div> <script src="scripts/three.js"></script><!--DL this from official site--> <script src="scripts/app.js"></script> </body> </html>
Accessing it from Chrome Console as mentioned above:
var a = example.scene.getObjectByName('box'); a.position.x = 10; a.position.set(0,2,2);
- Degrees to radians formula: radians = degrees *pi/180
- Meshes – geometry + material
- use stats.js
- different existing controls: FlyControls.js, OrbitControls.js
- Collision detection
- Raycasting – returns objects that are on some other objects “ray” and Box3.
- Physics: PhysiJS is a wrapper for Ammo.js which is a port of C++ Bullet
- Supports gravity, mass property, friction, restitution (bounciness)
Cool 3D frogger game made by the author: https://github.com/alexmackey/threeJsFrogger
Leave a Comment