Adding AdMob to Ionic framework application step by step
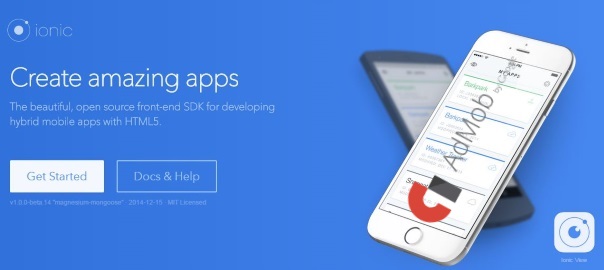
In case you’re looking for a way to implement Google AdMob ads in Ionic framework 3, then check out this tutorial: How to make money with Google AdMob ads in Ionic framework 3.
If you want, you can take a look at the screencast of this tutorial. This is actually my first screencast blog post so go easy on me 🙂
I’ve been searching for a start to end tutorial on this but with no luck. Now, when I finally figured out how to use it, I’m documenting the exact steps which I did in order to get the AdMob working inside my Ionic app. You can fork the project from GitHub or download and test the .apk file on your device.
I broke down the steps in two parts: AdMob settings and Ionic settings.
AdMob settings
Let’s start with AdMob settings:
- Sign in /Sign up for AdMob at https://www.google.com/admob/
- Click the Monetize new app button:
- If your app is not yet published you can add it manually:
- Create new tracking ID:
- Configure the adds type, size, placement, style, name:
- You can read additional info on how to implement GA and AdMob, but for now let’s just click Done:
- You will now see the following similar screen:
The most important thing to note here is this Ad unit ID, which in my test case is
ca-app-pub-7957971173858308/3599533362
Ionic settings
I’m counting on the fact that you know how to use Ionic since you’re looking for a specific topic like this, so am going skip the part of actually explaining how to use the Ionic cli. Ok, enough chit-chat, now I’m going to show you the steps on how to implement AdMob in your Ionic project:
- Start a new Ionic blank project:
ionic create IonicAdMobTest blank
- Add the platform for which you want to build the application:
-
ionic platform add android
In case you’re developing on a Mac, you can also choose to add:
ionic platform add ios
- Add the cordova-plugin-admob plugin by entering the following command in your command prompt when in the root folder of your project:
cordova plugin add com.rjfun.cordova.plugin.admob
As a side note, you can always check the list of all installed plugins by executing:
cordova plugin list
- Add the following code to your app.js file (located in the www folder) inside the .run function:
.run(function($ionicPlatform) { $ionicPlatform.ready(function() { // Hide the accessory bar by default (remove this to show the accessory bar above the keyboard // for form inputs) if (window.cordova && window.cordova.plugins.Keyboard) { cordova.plugins.Keyboard.hideKeyboardAccessoryBar(true); } if (window.StatusBar) { // org.apache.cordova.statusbar required StatusBar.styleDefault(); } if(window.plugins && window.plugins.AdMob) { var admob_key = device.platform == "Android" ? "ca-app-pub-7957971173858308/3666912163" : "ca-app-pub-7957971173858308/3666912163"; var admob = window.plugins.AdMob; admob.createBannerView( { 'publisherId': admob_key, 'adSize': admob.AD_SIZE.BANNER, 'bannerAtTop': false }, function() { admob.requestAd( { 'isTesting': false }, function() { admob.showAd(true); }, function() { console.log('failed to request ad'); } ); }, function() { console.log('failed to create banner view'); } ); } }); })
Of course, change it according to your own admob_key which you obtained in the first part (step 8).
- Start the emulator by running:
ionic build ios && ionic emulate ios //or, for android ionic build android && ionic emulate android
- and you should get the following screen in your emulated application:
- You can clone the project from GitHub, or download the .apk (unzip needed) file and test on your Android device.
That’s all folks, hope this helps someone by saving time on endless testing as I did :/
Thank you for this Tutorial, this is the one that turned to be useful for me, however i have a question, How to use this code to integrate a button for Interstitial Ads? Thanks!
Hey Rolando,
I’m glad to hear it was useful to you. While creating an ad widget, just select the interstitial option and you’ll get the different code which you can then use in the code I provided.
hi , thanks for your work ,, i make all this step on my app ionic but the ads not working , mean not show .
thanks
its all working on ios emulator but not working on ionic lab or ionic serve -l. wonder why, please help
That’s because plugins like that don’t work in lab or via serve – for them you need to use simulator or the real device.
great post, very helpfull
but how to put Interstitial admob? please
Thanks
Thanks, I’m glad it was helpful.
Take a look if this blog post I wrote on the subject helps you: http://www.nikola-breznjak.com/blog/stack-overflow/show-interstitial-ad-via-admob-in-ionic-every-2-minutes/
Hi Nikola,
Can i use admob on bottom together with the device buttons or google have any policy for this?
https://www.numetriclabz.com/wp-content/uploads/2016/02/14.jpg
I can use like this or it need a margin?
Nice tutorial.
Hi Felipe,
I have it like this. I’ve seen other apps have it like this – so I guess you’re fine by using it like this.
Thanks,
Nikola
Hi Nikola-breznjak
I am working in ios app, tried to add the plugin, but it displays
“Error: Failed to fetch plugin https://registry.npmjs.org/com.rjfun.cordova.plugin.admob via git.
Either there is a connection problems, or plugin spec is incorrect:
Error: git: Command failed with exit code 128 Error output:
Cloning into ‘/var/folders/85/b2lskfld5md_k3_ygjhydcb40000gn/T/git/1486572875605’…
fatal: repository ‘https://registry.npmjs.org/com.rjfun.cordova.plugin.admob/’ not found”
Please share the updated plugin link to install in my ios app.
Hey, please try this link: http://ngcordova.com/docs/plugins/adMob/
Thanks for you article. Can do this with React Native ?
Yes, it seems you can: https://github.com/sbugert/react-native-admob
Hi, I followed your steps but the banner is showing blank black area with no ads appearing. Any solutions please
Can you please try again? It takes some time for this to propagate? If that still doesn’t work, just in case, I would check if you added your IDs and not copied mine?
Hi Nikola! Great blog and thanks for your help. I have a similar problem to Tantan – what should the publisherID be set as? I’ve used all the logical IDs I have on me – I was wondering if you could let me know what should be set here.
Thanks!
See in the tutorial where I say “The most important thing to note here is this Ad unit ID, which in my test case is
ca-app-pub-7957971173858308/3599533362”. Take a look at the image above. It should help you figure out what is your key. Feel free to ping me if you need any further help. ?
Nikola, got it to work!
It turned out to be a problem with AdMob itself (there was a campaign setup). Once this campaign was deleted, I was able to add Google’s test credentials (similar to yours), the demo ad would then show.
Your tutorial was quite simply brilliant! Cheers mate.
Hey Rye, awesome, I’m glad you got it working! Sure, no problems – ping me if you get stuck!
sir how to add a videoreward ads in ionic-v1
Hey Junaid,
What have you tried so far?
Can’t find app.js ?? I have tried it other ways but it always shows “Plugin not installed… add plugin” But I have already installed the plugin. I have tried everything: deleting node_modules, plugins, platforms, reinstalling all of them. Tried to remove and add that particular plugin again..nothing worked! Please help
What version of Ionic are you using?
Hi! I’m trying to implement this tutorial (I hope it’s not too late to do it) but the Ad isn’t showing, even installing the APK that you provide us :(.
Do you know if something changed?
I appreciate your response so much. Thank you!
When we are merge above code then if I open the app it will be automatically minimized. What should I do?